Detecting C# Security Vulnerabilities with SonarQube
SonarQube is a tool that performs static code analysis on your codebase to detect bugs, code smells and security vulnerabilities early in the development process—without running the code. It helps maintain code quality and enforce best practices during development. On the other hand, dynamic analysis tools look at how your code behaves while it’s running, detecting problems like performance issues, memory leaks, security risks, etc.
The SonarQube documentation describes a vulnerability as:
A point in your code that's open to attack.
This blog post will guide you through using SonarQube to identify security vulnerabilities in a simple C# console app, highlight an example of vulnerable code, and then show how to fix it to close the security loophole.
Step 1 - Run SonarQube with Docker
Let’s spin up SonarQube locally using Docker Compose:
version: "3"
services:
sonarqube:
image: sonarqube:latest
container_name: sonarqube
ports:
- "9090:9000"
environment:
- SONAR_ES_BOOTSTRAP_CHECKS_DISABLE=true
volumes:
- sonarqube_data:/opt/sonarqube/data
- sonarqube_logs:/opt/sonarqube/logs
- sonarqube_extensions:/opt/sonarqube/extensions
volumes:
sonarqube_data:
sonarqube_logs:
sonarqube_extensions:
Run it:
docker-compose up -d
Then go to:
http://localhost:9090
Log credentials:
- Username:
admin
- Password:
admin
Step 2 - Create a Project and Generate a Token
Create a Project in SonarQube
Click Create a local project.
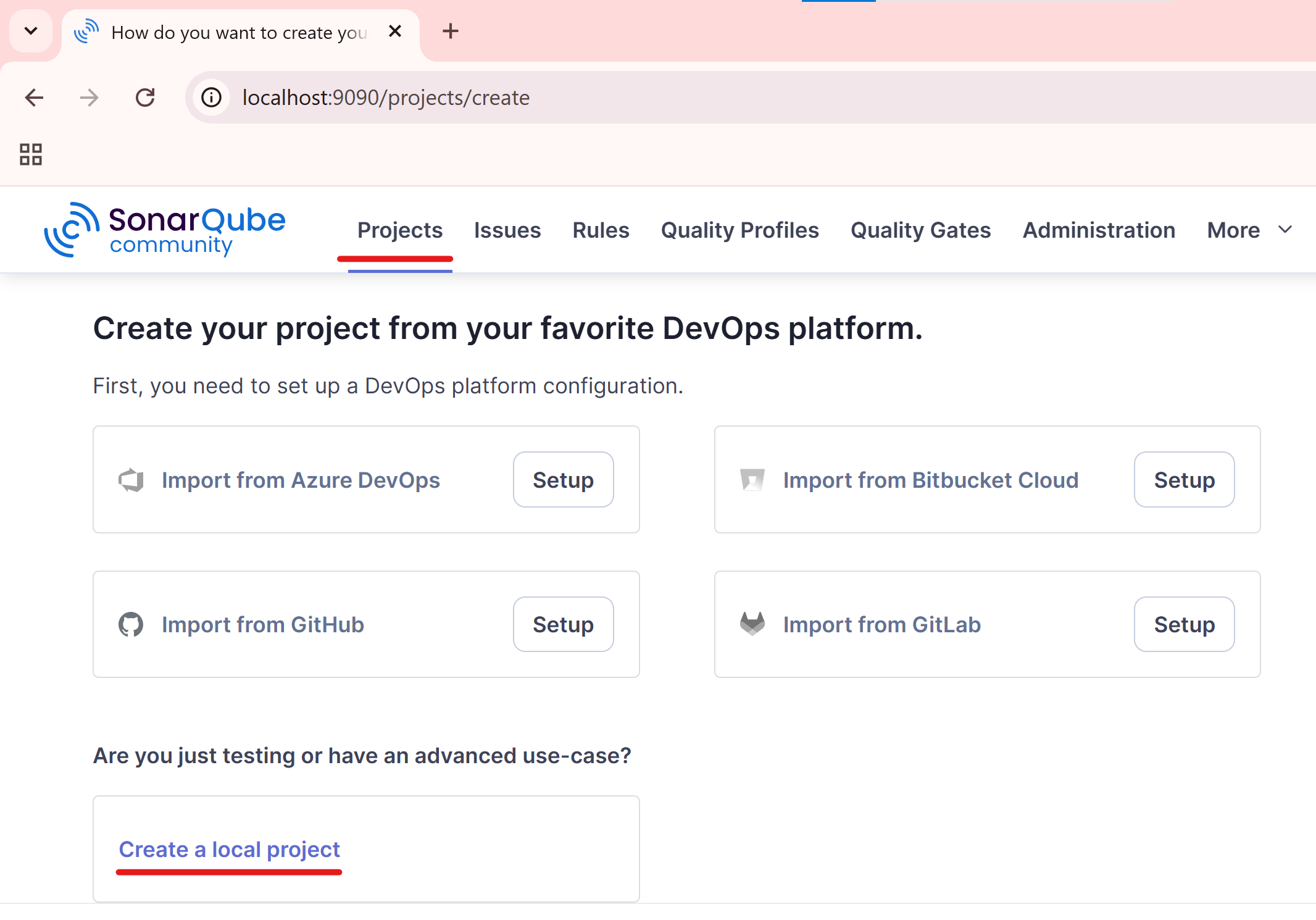
Enter a project name e.g. VulnerableApp.
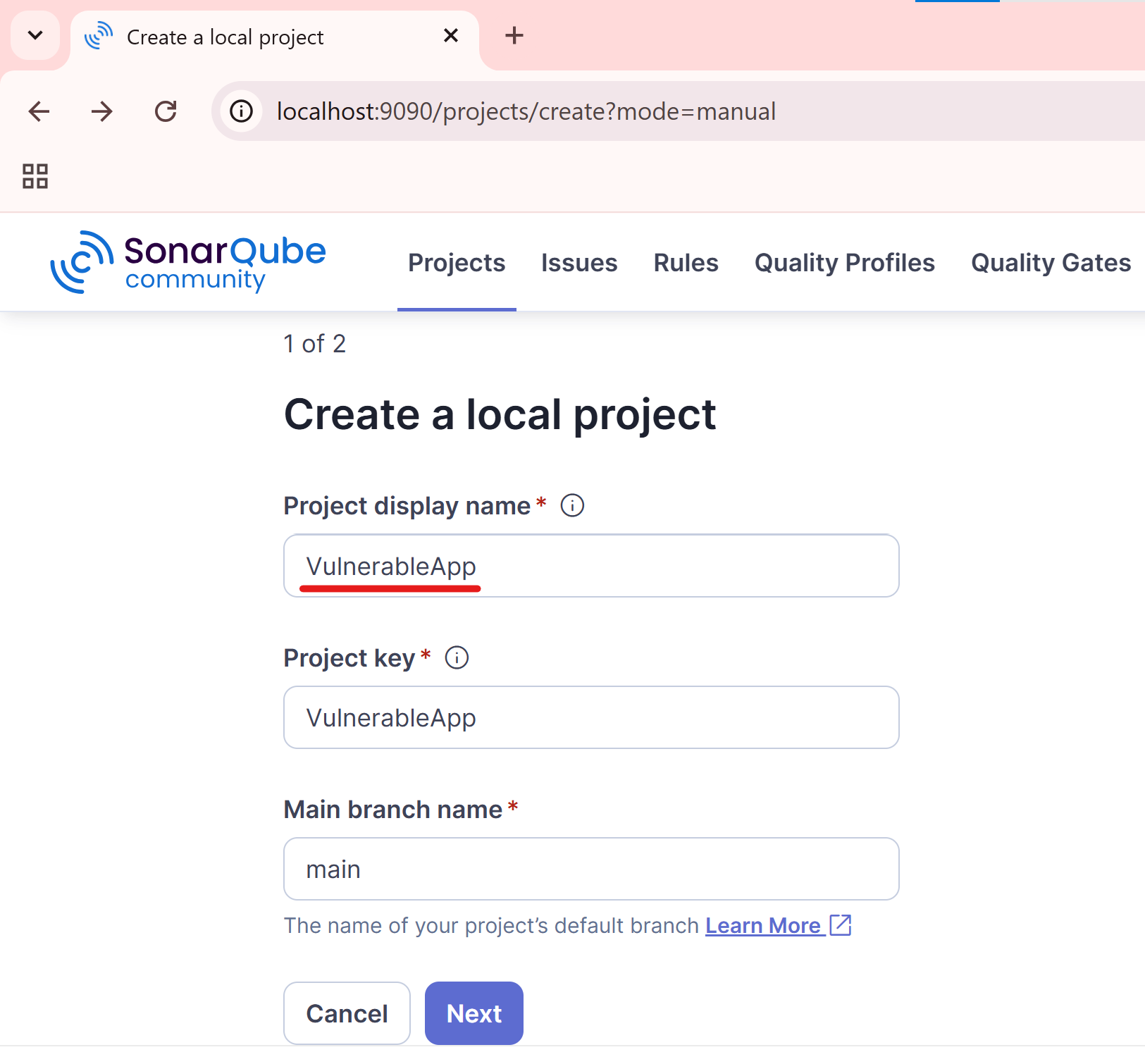
Use the global settings. This option applies system-wide defaults to a project unless overridden locally.
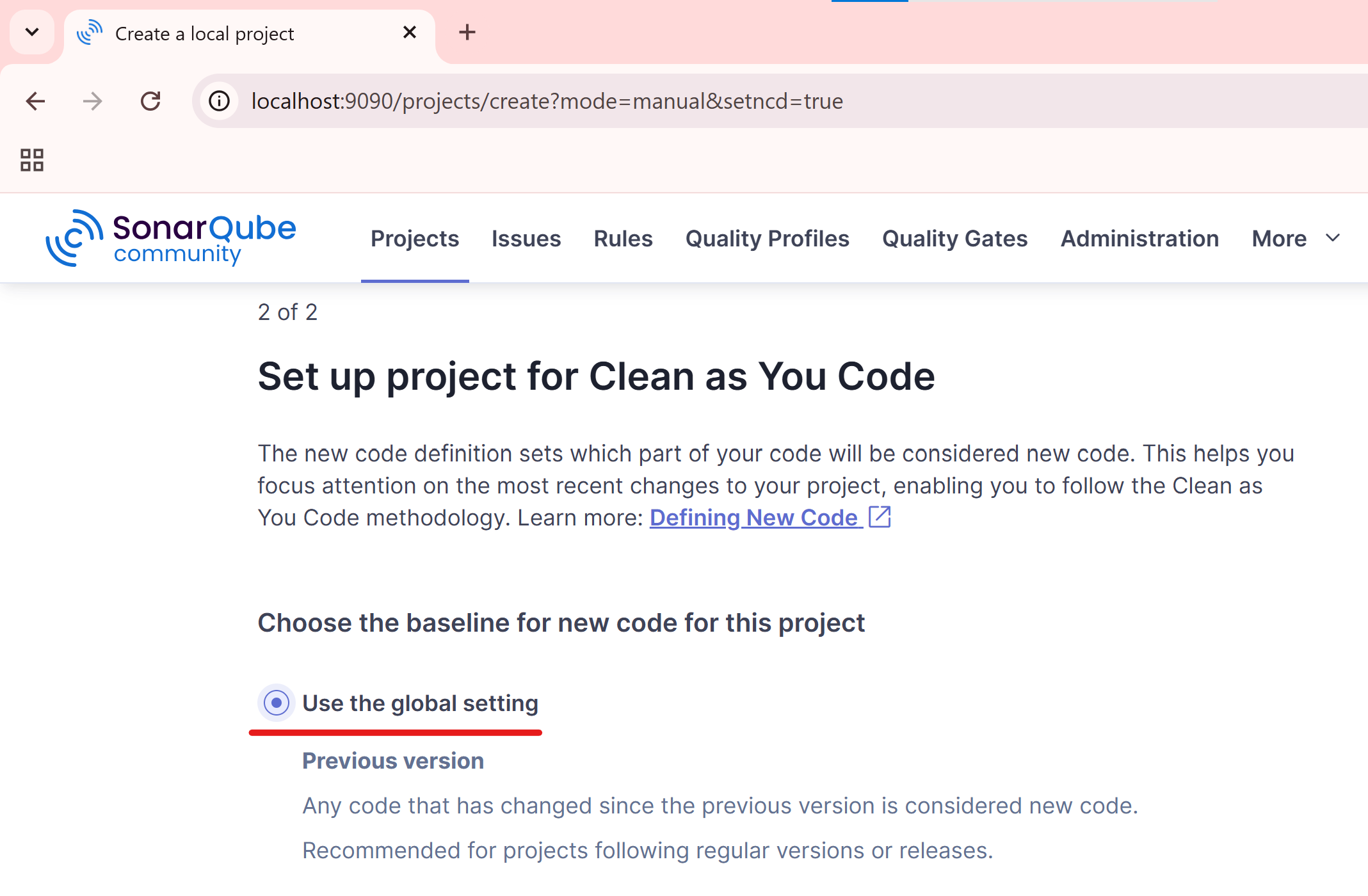
Generate a Token
Click your profile icon (top-right corner).
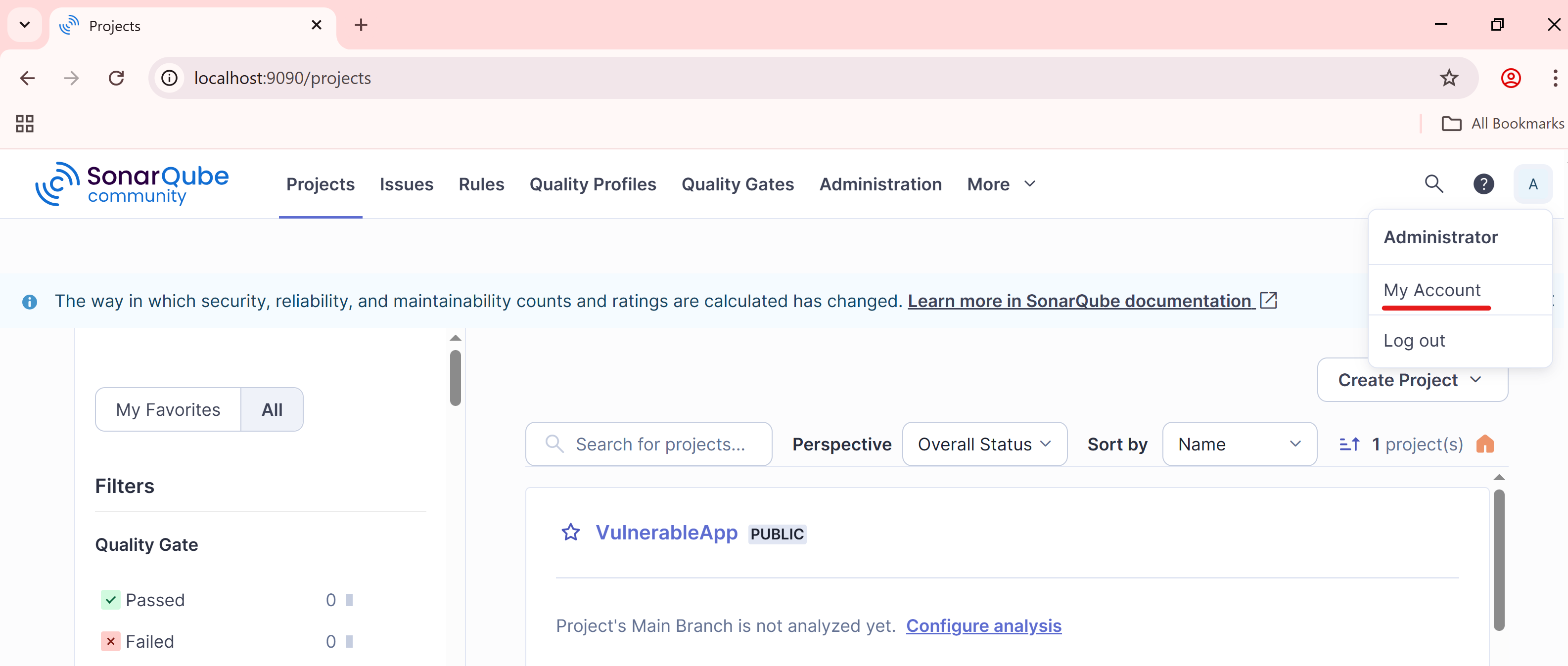
Select My Account > Security tab.
In the Generate Tokens section:
- Enter a Token Name (e.g.,
VulnerableAppToken
). - Select Project Analysis Token as the Token Type
- Select the Project as VulnerableApp
- Click Generate.
- Copy the token immediately (you won’t be able to see it again).
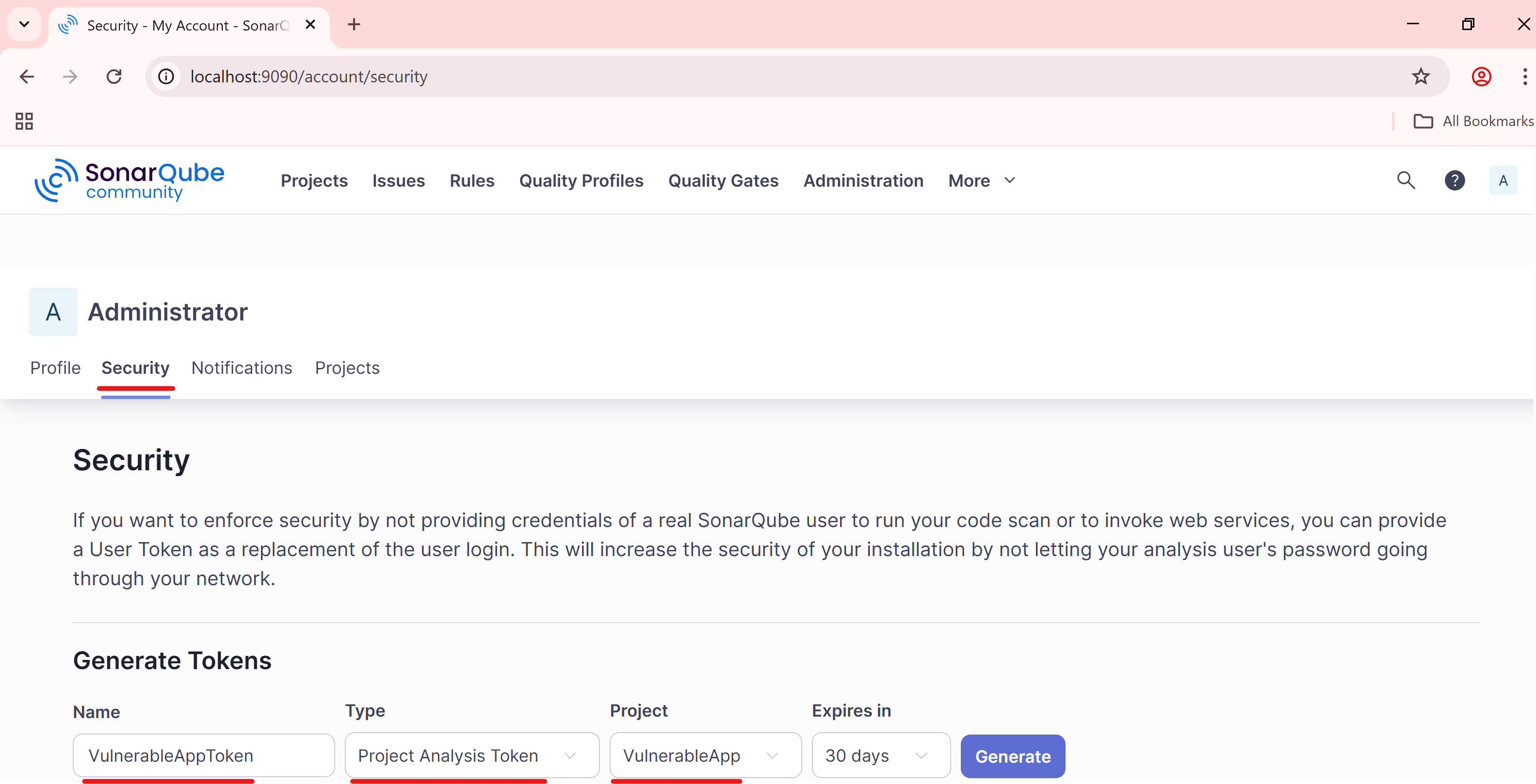
We will use the token when running the scanner.
Step 3 - Create a Vulnerable C# Console App
Now let’s create a simple app that includes a real security vulnerability. The code uses the MD5 hash algorithm to hash sensitive data. MD5 is insecure due to its vulnerability to collision attacks, allowing attackers to create different inputs with the same hash. It should not be used for securing sensitive data like passwords or tokens.
using System.Security.Cryptography;
using System.Text;
using (MD5 md5 = MD5.Create())
{
byte[] inputBytes = Encoding.UTF8.GetBytes("sensitiveData");
var hash = md5.ComputeHash(inputBytes);
Console.WriteLine(Convert.ToBase64String(hash).ToLower());
}
Step 4 - Download and install SonarScanner for .NET Core
dotnet tool install --global dotnet-sonarscanner
Step 5 - Scan the Code with SonarQube
Run:
dotnet sonarscanner begin /k:"VulnerableApp" /d:sonar.host.url="http://localhost:9090" /d:sonar.login="sqp_93052c0e5b5de289ab18865aa7eabc76d3c9426c"
dotnet sonarscanner begin: Starts the analysis, specifying the project key, SonarQube server URL, and authentication token.
dotnet build
dotnet build: Builds the project.
dotnet sonarscanner end /d:sonar.login="sqp_93052c0e5b5de289ab18865aa7eabc76d3c9426c"
dotnet sonarscanner end: Completes the analysis and uploads the results to the SonarQube server using the authentication token.
Step 6 - View the Results
Head back to the SonarQube UI and open the VulnerableApp project. You’ll notice that SonarQube has identified the use of a weak hash algorithm—listed under the Security Hotspots section.
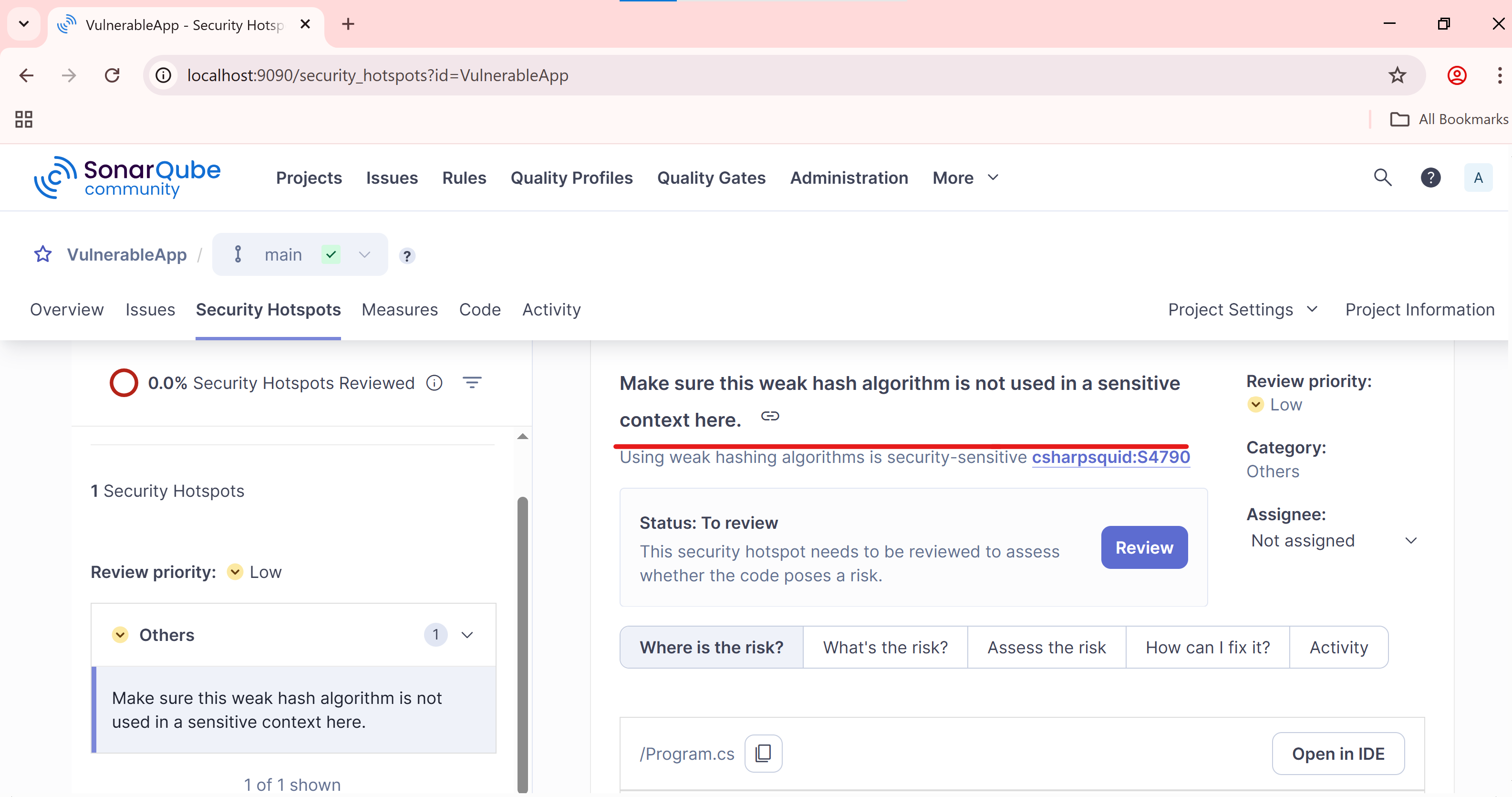
Step 7 - Fix and Rescan
SonarQube recommends addressing this vulnerability by using a stronger hashing algorithm—such as SHA-256. SHA-256 offers enhanced security through its 256-bit hash, making it far more resistant to collisions and brute-force attacks.
using System.Security.Cryptography;
using System.Text;
using (SHA256 sha256 = SHA256.Create())
{
byte[] inputBytes = Encoding.UTF8.GetBytes("sensitiveData");
var hash = sha256.ComputeHash(inputBytes);
Console.WriteLine(Convert.ToBase64String(hash).ToLower());
}
Rebuild the project and run SonarScanner again. The vulnerability is resolved.
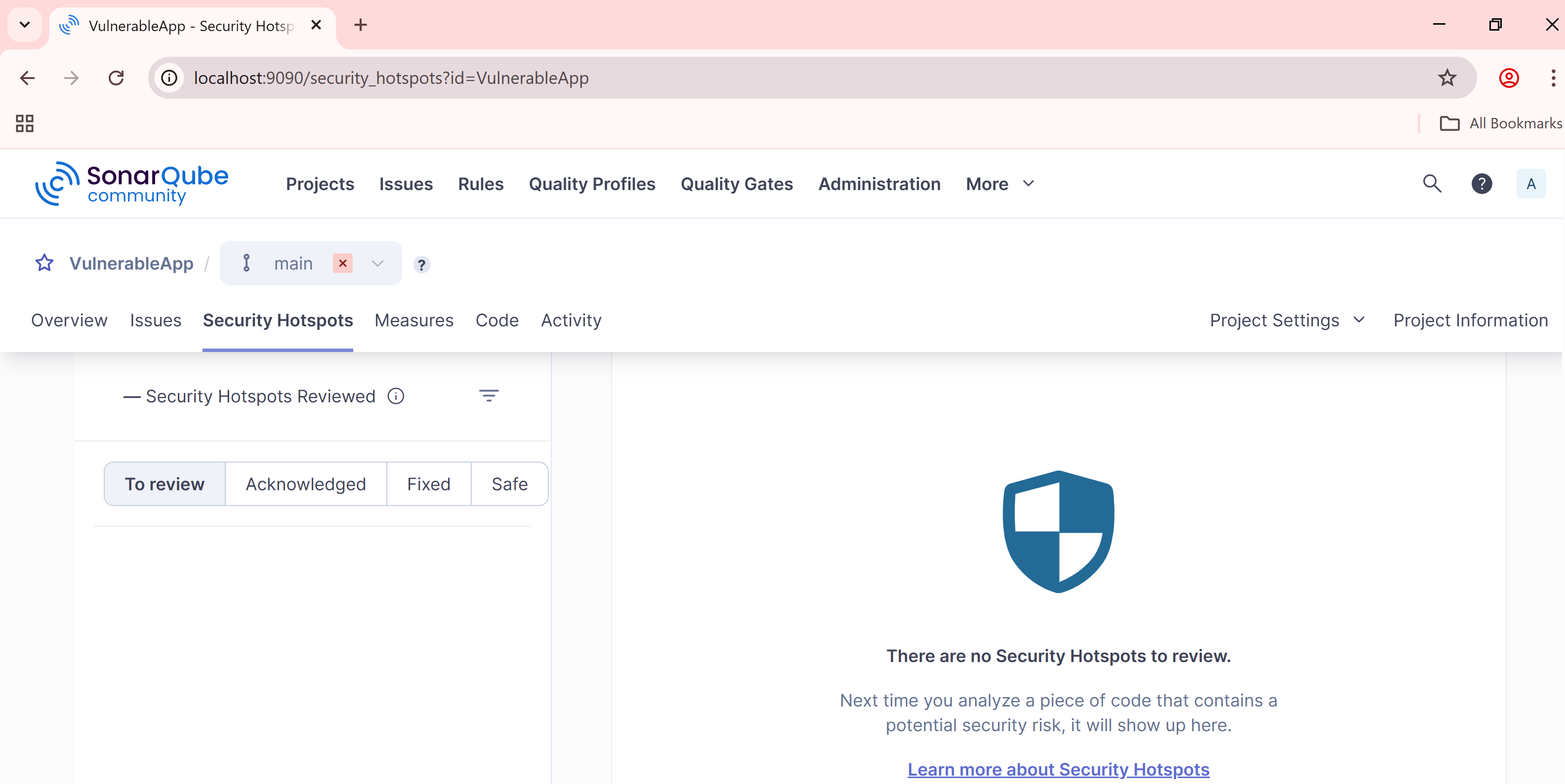